Overview of all Endpoints
The API base URL is: https://api.deepva.com/api/v1
Perform CRUD operations on the core-resources in REST style.
Visual Mining Jobs
Visual Mining Jobs are available on the Jobs-Endpoint.
/Jobs
GET /jobs/
Retrieve a list of Visual Mining Jobs
Returns: Pagination object with list of Job objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
search
- List jobs by id and tag
tag
- List jobs by tag
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/jobs/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/jobs/",
headers=headers)
if response.status_code == 200:
jobs = response.json()
print(jobs)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 1,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/datasets/?limit=5&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/?limit=5&offset=0",
"data": [
{
"id": "6b5195d6-cc05-4a99-a293-8d73be0aa37f",
"tag": "",
"state": "completed",
"progress": 1.0,
"duration": 0.2,
"time_created": "2019-08-12 08:14:28.430144",
"time_started": "2019-08-12 08:14:29.510771",
"time_completed": "2019-08-12 08:14:29.530825",
"sources": [
"https://demo.deepva.com/demo1.jpg"
],
"modules": {
"object_scene_recognition": {
"model": "general"
}
},
"result": {
"detailed_link": "https://api.deepva.com/v1/jobs/6b5195d6-cc05-4a99-a293-8d73be0aa37f/detailed-results",
"summary": [
{
"source": "https://demo.deepva.com/demo1.jpg",
"media_type": "image",
"info": {},
"items": [
{
"type": "object",
"label": "Indoor"
},
{
"type": "object",
"label": "Chair"
},
{
"type": "object",
"label": "Furniture"
},
{
"type": "object",
"label": "Cushion"
}
]
}
]
}
]
}
GET /jobs/{JOB_ID}/
Retrieves a single Visual Mining Jobs by its ID
Returns: Job object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/jobs/{job_id}/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/jobs/{job_id}/",
headers=headers)
if response.status_code == 200:
job = response.json()
print(job)
else:
print(f"Error: {response.status_code}")
Example response:
{
"id": "6b5195d6-cc05-4a99-a293-8d73be0aa37f",
"tag": "",
"state": "completed",
"progress": 1.0,
"duration": 0.2,
"time_created": "2019-08-12 08:14:28.430144",
"time_started": "2019-08-12 08:14:29.510771",
"time_completed": "2019-08-12 08:14:29.530825",
"sources": [
"https://demo.deepva.com/demo1.jpg"
],
"modules": {
"object_scene_recognition": {
"model": "general"
}
},
"result": {
"detailed_link": "https://api.deepva.com/v1/jobs/6b5195d6-cc05-4a99-a293-8d73be0aa37f/detailed-results",
"summary": [
{
"source": "https://demo.deepva.com/demo1.jpg",
"media_type": "image",
"info": {},
"items": [
{
"type": "object",
"label": "Indoor"
},
{
"type": "object",
"label": "Chair"
},
{
"type": "object",
"label": "Furniture"
},
{
"type": "object",
"label": "Cushion"
}
]
}
]
}
POST /jobs/
Creates a new Visual Mining Job
Returns: Job object that has been created
Example request:
curl -d '{"sources": ["https://demo.deepva.com/demo1.jpg"], "modules": {"object_scene_recognition": {"model": "general"}}}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/jobs/
# coming soon
Example response:
{
"id": "3ad21bb4-774e-45e0-8c1f-15ec42037644",
"tag": "",
"state": "waiting",
"errors": [],
"progress": 0,
"duration": 0,
"time_created": "2020-08-14 14:21:20.777910",
"time_started": null,
"time_completed": null,
"sources": [
"https://demo.deepva.com/demo1.jpg"
],
"modules": {
"object_scene_recognition": {
"model": "general",
"state": "waiting"
}
},
"result": {
"detailed_link": "https://api.deepva.com/v1/jobs/3ad21bb4-774e-45e0-8c1f-15ec42037644/detailed-results/",
"summary": []
}
}
The Job has just been created. The state
is "waiting" becasue it waits for processing by DeepVA. There is no result available yet. result.summary
is empty.
You can request the Job by its ID and check the state: https://api.deepva.com/v1/jobs/3ad21bb4-774e-45e0-8c1f-15ec42037644/
DELETE /jobs/{JOB_ID}/
Deletes and aborts an existing Visual Mining Job
Returns: Message saying that the job has been deleted
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v1/jobs/{job_id}/
# coming soon
Example response:
{
"message": "Job has been deleted"
}
GET /jobs/{JOB_ID}/artifacts/
Retrieve a list of all artifacts of a job
Returns: Pagination object with list of Artifact objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/jobs/{job_id}/artifacts
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/jobs/{job_id}/artifacts",
headers=headers)
if response.status_code == 200:
artifacts = response.json()
print(artifacts)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 1,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"id": "7103f3f6-3aaf-44bc-aef3-661dac15b32a",
"time_created": "2023-03-16T16:12:35.853632Z",
"time_expiration": "2023-03-23T16:12:35.853319Z",
"filename": "cd661240-84df-4d93-b007-934d9d6b1b64.png",
"mime_type": null
}
]
}
GET /jobs/{job_id}/artifacts/{ARTIFACT_ID}/file/
Retrieve an artifact file
Returns: Artifact file
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/jobs/{job_id}/artifacts/{artifact_id}/file
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/jobs/{job_id}/artifacts/{artifact_id}/file",
headers=headers)
if response.status_code == 200:
artifact = response.content
print(artifact)
else:
print(f"Error: {response.status_code}")
/Summarized results
To retrieve a summary of the job result you need to request the sub-endpoint: summarized-results
.
GET /jobs/{JOB_ID}/summarized-results/
Retrieve summarized results of a single Visual Mining Jobs by its job ID
Returns: Pagination object with list of SummarizedResult objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/jobs/{job_id}/summarized-results/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/jobs/{job_id}/summarized-results/",
headers=headers)
if response.status_code == 200:
results = response.json()
print(results)
else:
print(f"Error: {response.status_code}")
Example response for the result of the Advanced Diversity Analysis module:
{
"total": 5,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/jobs/f662463f-b440-4901-b032-6e6b202955f6/summarized-results/?limit=10&offset=10",
"prev": "http://api.deepva.com/api/v1/jobs/f662463f-b440-4901-b032-6e6b202955f6/summarized-results/?limit=10&offset=0",
"data": [
{
"id": "a143d847-49ed-453e-a2ba-93e03a63a810",
"source": "https://www.youtube.com/watch?v=wxN1T1uxQ2g",
"module": "advanced_diversity_analysis",
"meta": {
"female": 1,
"male": 4
},
"type": "gender_count"
},
{
"id": "42440471-8350-4529-bc18-2e99a0e3d1fc",
"source": "https://www.youtube.com/watch?v=wxN1T1uxQ2g",
"module": "advanced_diversity_analysis",
"meta": {
"30-40": 2,
"50-60": 1,
"60-70": 1,
"40-50": 1
},
"type": "age_count"
},
{
"id": "d3a9087d-61f9-4152-a26d-18c866b6b5bd",
"source": "https://www.youtube.com/watch?v=wxN1T1uxQ2g",
"module": "advanced_diversity_analysis",
"meta": {
"female_1": 26.0,
"male_1": 4.0,
"male_2": 2.5,
"male_3": 13.0,
"male_4": 4.5
},
"type": "person_screen_time"
},
{
"id": "d64bcc4d-1ce3-4232-b6c0-d28a02d25545",
"source": "https://www.youtube.com/watch?v=wxN1T1uxQ2g",
"module": "advanced_diversity_analysis",
"meta": {
"female": 26.0,
"male": 24.0
},
"type": "gender_screen_time"
},
{
"id": "ce8e3522-ba3d-4e8d-85ec-39f69e25cc22",
"source": "https://www.youtube.com/watch?v=wxN1T1uxQ2g",
"module": "advanced_diversity_analysis",
"meta": {
"30-40": 30.5,
"50-60": 4.0,
"60-70": 2.5,
"40-50": 13.0
},
"type": "age_screen_time"
}
]
}
/Detailed results
A Job object contains the result summary. For more detailed results (e.g. the position of identity or object in the video) you need to request the sub-endpoint: detailed-results
.
GET /jobs/{JOB_ID}/detailed-results/
Retrieve detailed results of a single Visual Mining Jobs by its job ID
Returns: Pagination object with list of DetailedResult objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/jobs/{job_id}/detailed-results/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/jobs/{job_id}/detailed-results/",
headers=headers)
if response.status_code == 200:
results = response.json()
print(results)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 4,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/jobs/21b61bd9-3d82-41a7-8cab-f154eaf6783c/detailed-results/?limit=10&offset=10",
"prev": "http://api.deepva.com/api/v1/jobs/21b61bd9-3d82-41a7-8cab-f154eaf6783c/detailed-results/?limit=10&offset=0",
"data": [
{
"media_type": "video",
"frame_start": 12,
"frame_end": 56,
"source": "storage://Fad9kJ0jSI3swC6N5hVp",
"module": "face_recognition",
"meta": {
"person": "Alexander Schwolow",
"mean_distance": 0.93,
"mean_similarity": 0.56
},
"time_start": 0.48,
"time_end": 2.24,
"tc_start": "00:00:12:00",
"tc_end": "00:00:56:00",
"detections": [
{
"frame_index": 12,
"type": "face_bbox",
"data": {
"x": 0.636,
"y": 0.165,
"w": 0.089,
"h": 0.226
}
},
{
"frame_index": 24,
"type": "face_bbox",
"data": {
"x": 0.632,
"y": 0.169,
"w": 0.088,
"h": 0.222
}
},
{
"frame_index": 36,
"type": "face_bbox",
"data": {
"x": 0.634,
"y": 0.157,
"w": 0.09,
"h": 0.235
}
},
{
"frame_index": 48,
"type": "face_bbox",
"data": {
"x": 0.639,
"y": 0.161,
"w": 0.089,
"h": 0.233
}
}
],
},
{
"media_type": "video",
"frame_start": 12,
"frame_end": 56,
"source": "storage://Fad9kJ0jSI3swC6N5hVp",
"module": "face_recognition",
"meta": {
"person": "Vincenzo Grifo",
"mean_distance": 0.88,
"mean_similarity": 0.61
},
"time_start": 0.48,
"time_end": 2.24,
"tc_start": "00:00:12:00",
"tc_end": "00:00:56:00",
"detections": [
{
"frame_index": 12,
"type": "face_bbox",
"data": {
"x": 0.831,
"y": 0.146,
"w": 0.095,
"h": 0.222
}
},
{
"frame_index": 24,
"type": "face_bbox",
"data": {
"x": 0.821,
"y": 0.139,
"w": 0.094,
"h": 0.213
}
},
{
"frame_index": 36,
"type": "face_bbox",
"data": {
"x": 0.824,
"y": 0.135,
"w": 0.094,
"h": 0.211
}
},
{
"frame_index": 48,
"type": "face_bbox",
"data": {
"x": 0.826,
"y": 0.133,
"w": 0.097,
"h": 0.211
}
}
]
},
{
"media_type": "video",
"frame_start": 468,
"frame_end": 668,
"source": "storage://Fad9kJ0jSI3swC6N5hVp",
"module": "face_recognition",
"meta": {
"person": "Julian Schuster",
"mean_distance": 0.89,
"mean_similarity": 0.6
},
"time_start": 18.72,
"time_end": 26.72,
"tc_start": "00:07:48:00",
"tc_end": "00:11:08:00",
"detections": [
{
"frame_index": 468,
"type": "face_bbox",
"data": {
"x": 0.546,
"y": 0.141,
"w": 0.219,
"h": 0.613
}
},
{
"frame_index": 480,
"type": "face_bbox",
"data": {
"x": 0.532,
"y": 0.146,
"w": 0.226,
"h": 0.617
}
},
{
"frame_index": 492,
"type": "face_bbox",
"data": {
"x": 0.561,
"y": 0.135,
"w": 0.222,
"h": 0.609
}
},
{
"frame_index": 504,
"type": "face_bbox",
"data": {
"x": 0.569,
"y": 0.143,
"w": 0.219,
"h": 0.604
}
},
{
"frame_index": 516,
"type": "face_bbox",
"data": {
"x": 0.554,
"y": 0.148,
"w": 0.22,
"h": 0.604
}
},
{
"frame_index": 528,
"type": "face_bbox",
"data": {
"x": 0.558,
"y": 0.131,
"w": 0.223,
"h": 0.617
}
},
{
"frame_index": 540,
"type": "face_bbox",
"data": {
"x": 0.578,
"y": 0.126,
"w": 0.22,
"h": 0.617
}
},
{
"frame_index": 552,
"type": "face_bbox",
"data": {
"x": 0.567,
"y": 0.139,
"w": 0.222,
"h": 0.62
}
},
{
"frame_index": 564,
"type": "face_bbox",
"data": {
"x": 0.553,
"y": 0.119,
"w": 0.221,
"h": 0.613
}
},
{
"frame_index": 576,
"type": "face_bbox",
"data": {
"x": 0.529,
"y": 0.137,
"w": 0.224,
"h": 0.613
}
},
{
"frame_index": 588,
"type": "face_bbox",
"data": {
"x": 0.576,
"y": 0.124,
"w": 0.215,
"h": 0.604
}
},
{
"frame_index": 600,
"type": "face_bbox",
"data": {
"x": 0.568,
"y": 0.128,
"w": 0.215,
"h": 0.589
}
},
{
"frame_index": 612,
"type": "face_bbox",
"data": {
"x": 0.56,
"y": 0.124,
"w": 0.218,
"h": 0.593
}
},
{
"frame_index": 624,
"type": "face_bbox",
"data": {
"x": 0.559,
"y": 0.107,
"w": 0.218,
"h": 0.602
}
},
{
"frame_index": 636,
"type": "face_bbox",
"data": {
"x": 0.571,
"y": 0.113,
"w": 0.219,
"h": 0.604
}
},
{
"frame_index": 648,
"type": "face_bbox",
"data": {
"x": 0.555,
"y": 0.124,
"w": 0.221,
"h": 0.602
}
},
{
"frame_index": 660,
"type": "face_bbox",
"data": {
"x": 0.546,
"y": 0.109,
"w": 0.215,
"h": 0.598
}
}
]
},
{
"media_type": "video",
"frame_start": 720,
"frame_end": 792,
"source": "storage://Fad9kJ0jSI3swC6N5hVp",
"module": "face_recognition",
"meta": {
"person": "unknown",
"closest_person": "Charlie Manuel",
"mean_distance": 1.05,
"mean_similarity": 0.45
},
"time_start": 28.8,
"time_end": 31.68,
"tc_start": "00:12:00:00",
"tc_end": "00:13:12:00",
"detections": [
{
"frame_index": 720,
"type": "face_bbox",
"data": {
"x": 0.499,
"y": 0.281,
"w": 0.104,
"h": 0.3
}
},
{
"frame_index": 732,
"type": "face_bbox",
"data": {
"x": 0.55,
"y": 0.241,
"w": 0.127,
"h": 0.356
}
},
{
"frame_index": 744,
"type": "face_bbox",
"data": {
"x": 0.558,
"y": 0.248,
"w": 0.134,
"h": 0.343
}
},
{
"frame_index": 756,
"type": "face_bbox",
"data": {
"x": 0.53,
"y": 0.224,
"w": 0.147,
"h": 0.365
}
},
{
"frame_index": 768,
"type": "face_bbox",
"data": {
"x": 0.492,
"y": 0.183,
"w": 0.159,
"h": 0.38
}
},
{
"frame_index": 780,
"type": "face_bbox",
"data": {
"x": 0.457,
"y": 0.172,
"w": 0.152,
"h": 0.365
}
}
]
}
]
}
Dataset Management
/Datasets
GET /datasets/
Retrieve a list of Datset objects.
Returns: Pagination object with list of Dataset objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/
# coming soon
Example response:
{
"total": 8,
"offset": 0,
"limit": 3,
"next": "http://api.deepva.com/api/v1/datasets/?limit=10&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/?limit=10&offset=0",
"data": [
{
"id": "015308de-f432-430b-b659-208e16fd5559",
"name": "Demo",
"description": "This is a dataset",
"type": "face",
"time_created": "2020-02-06 17:35:46.955000",
"time_updated": "2020-02-06 17:35:46.955000",
"number_of_classes": 25,
"number_of_active_classes": 25,
"number_of_images": 144,
"number_of_active_images": 144,
"preview_images": [
"storage://0jmGBdonP9fBQjDdCoDi",
"storage://vcK8yLNYMUoWVW3IfnGS",
"storage://2x2Oeim8Ur3YzURmLSoU"
],
"evaluation_result": {}
},
{
"id": "1db75c6b-0767-4e2f-93d1-dca58f25e588",
"name": "Test",
"description": "This is a test dataset",
"type": "face",
"time_created": "2020-03-29 14:19:30.059000",
"time_updated": "2020-03-29 14:19:30.059000",
"number_of_classes": 7,
"number_of_active_classes": 7,
"number_of_images": 26,
"number_of_active_images": 26,
"preview_images": [
"storage://Xm88Y6BNjRHX5IeofeeO",
"storage://OeidQke7tgLzY5yDHC7Z",
"storage://3Qgifdu7dYxAFcDoiTQd"
],
"evaluation_result": {}
},
{
"id": "d9e5e1ae-23e8-4d41-b11a-46558776a139",
"name": "My Dataset",
"description": "This is a dataset",
"type": "face",
"time_created": "2020-03-31 05:47:01.513000",
"time_updated": "2020-03-31 05:47:01.513000",
"number_of_classes": 3,
"number_of_active_classes": 3,
"number_of_images": 13,
"number_of_active_images": 13,
"preview_images": [
"storage://mvbBcUz84a7RhahdFtU2",
"storage://ctIbUq0XZzs38aiU6ITR",
"storage://owWSFFjz5upPliDNd5GP"
],
"evaluation_result": {}
}
]
}
GET /datasets/{DATASET_ID}/
Retrieves a single Dataset object by its ID
Returns: Dataset object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/
# coming soon
Example response:
{
"id": "8dadd217-334f-4b45-9208-202310cedf68",
"name": "My New Dataset",
"description": "This is a dataset",
"type": "face",
"time_created": "2020-08-14 17:49:46.896000",
"time_updated": "2020-08-14 17:49:46.896000",
"number_of_classes": 1,
"number_of_active_classes": 1,
"number_of_images": 0,
"number_of_active_images": 0,
"preview_images": [],
"evaluation_result": {}
}
POST /datasets/
Creates a new Dataset
Returns: Dataset object that has been created
Example request:
curl -d '{"name": "My New Dataset", "description": "This is a dataset", "type": "face"}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/datasets/
# coming soon
Example response:
{
"id": "b30a68e4-1853-4e83-89fb-5d135415ead3",
"name": "My New Dataset",
"description": "This is a dataset",
"type": "face",
"time_created": "2020-08-26 08:23:21.303951",
"time_updated": "2020-08-26 08:23:21.303959",
"number_of_classes": 0,
"number_of_active_classes": 0,
"number_of_images": 0,
"number_of_active_images": 0,
"preview_images": [],
"evaluation_result": {}
}
DELETE /datasets/{DATASET_ID}/
Deletes a Dataset
Returns: Message saying that the dataset has been deleted
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v1/datasets/{DATASET_ID}/
# coming soon
Example response:
{
"message": "Dataset has been deleted"
}
/Classes
GET /datasets/{DATASET_ID}/classes/
Retrieve a list of Class objects.
Returns: Pagination object with list of Class objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/
# coming soon
Example response:
{
"total": 1,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/datasets/8dadd217-334f-4b45-9208-202310cedf68/classes/?limit=10&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/8dadd217-334f-4b45-9208-202310cedf68/classes/?limit=10&offset=0",
"data": [
{
"id": "ab6c8b9a-2946-475b-89c2-f94e94d3e7d1",
"reference": "bc2c4033-7b16-4122-abf9-1d4907c4599d",
"label": "John Doe",
"preview_image": {
"id": "feb5a6ca-7eb2-4151-87a6-760ba50dcdb0",
"file_uri": "storage://jRsMJzpOkC3Yk59hKEIA",
"time_created": "2020-08-14 18:06:35.556000",
"time_updated": "2020-08-14 18:06:35.556000",
"active": true,
"notes": "",
"issue": -1,
"is_auto_generated": false,
"evaluation_result": {}
},
"time_created": "2020-08-14 17:59:15.096000",
"time_updated": "2020-08-14 18:06:35.558000",
"issues": [],
"number_of_images": 1,
"active": true,
"notes": "",
"expiration_date": null,
"custom_fields": [],
"level": -1,
"evaluation_result": {}
}
]
}
GET /datasets/{DATASET_ID}/classes/{CLASS_ID}/
Retrieves a single Class object by its ID
Returns: Class object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{class_id}/
# coming soon
Example response:
{
"id": "ab6c8b9a-2946-475b-89c2-f94e94d3e7d1",
"reference": "bc2c4033-7b16-4122-abf9-1d4907c4599d",
"label": "John Doe",
"preview_image": {
"id": "feb5a6ca-7eb2-4151-87a6-760ba50dcdb0",
"file_uri": "storage://jRsMJzpOkC3Yk59hKEIA",
"time_created": "2020-08-14 18:06:35.556000",
"time_updated": "2020-08-14 18:06:35.556000",
"active": true,
"notes": "",
"issue": -1,
"is_auto_generated": false,
"evaluation_result": {}
},
"time_created": "2020-08-14 17:59:15.096000",
"time_updated": "2020-08-14 18:06:35.558000",
"issues": [],
"number_of_images": 1,
"active": true,
"notes": "",
"expiration_date": null,
"custom_fields": [],
"level": -1,
"evaluation_result": {}
}
POST /datasets/{DATASET_ID}/classes/
Creates a new Class
Returns: Class object that has been created
Example request:
curl -d '{"label": "John Doe"}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/
# coming soon
Example response:
{
"id": "ab6c8b9a-2946-475b-89c2-f94e94d3e7d1",
"reference": "bc2c4033-7b16-4122-abf9-1d4907c4599d",
"label": "John Doe",
"preview_image": null,
"time_created": "2020-08-14 17:59:15.096000",
"time_updated": "2020-08-14 17:59:15.096000",
"issues": [],
"number_of_images": 1,
"active": true,
"notes": "",
"expiration_date": null,
"custom_fields": [],
"level": -1,
"evaluation_result": {}
}
DELETE /datasets/{DATASET_ID}/classes/{CLASS_ID}/
Deletes a Class
Returns: Message saying that the class has been deleted
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v1/datasets/{DATASET_ID}/classes/{CLASS_ID}/
# coming soon
Example response:
{
"message": "Class has been deleted"
}
Clustering
GET /datasets/{DATASET_ID}/classes/{CLASS_ID}/clusters/
Retrieve a list of Cluster objects. Clusters are available when Dataset Evaluation was triggered.
Returns: Pagination object with list of Cluster objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{class_id}/clusters/
# coming soon
Example response:
{
"total": 3,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/datasets/9538e44c-6f30-40b7-8d7c-73bda1d41a9e/class/3d08d7c2-6e15-4a48-9cbd-c04dc257b4f8/clusters/?limit=5&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/9538e44c-6f30-40b7-8d7c-73bda1d41a9e/class/3d08d7c2-6e15-4a48-9cbd-c04dc257b4f8/clusters/?limit=5&offset=0",
"data": [
{
"name": "cluster_main",
"preview_images":[
"storage://V4Ic75h6RYqKXK9TkkDw",
"storage://t7r2deRbEW8SxZy3oHyX",
"storage://IQuHT85doYr8jZOV3AkJ",
"storage://2n4pDh30LdyOOwhe0qyW"
]
},
{
"name": "cluster_1",
"preview_images":[
"storage://n5etIg75HHrU10smd6iF",
"storage://0tuoONKriUE9FYTnHFz9",
"storage://D22PZOps8OrXUbWA0IYh",
"storage://7gmS7dDLsf4EDhPiJYoS"
]
},
{
"name": "outlier",
"preview_images":[
"storage://v6oPWj4NYhEWTKBRnMnc"
]
}
]
}
GET /datasets/{DATASET_ID}/classes/{CLASS_ID}/clusters/{CLUSTER_NAME}/
Retrieves a single Cluster object by its cluster name.
Returns: Cluster object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{class_id}/clusters/cluster_main/
# coming soon
Example response:
{
"name": "cluster_main",
"preview_images":[
"storage://V4Ic75h6RYqKXK9TkkDw",
"storage://t7r2deRbEW8SxZy3oHyX",
"storage://IQuHT85doYr8jZOV3AkJ",
"storage://2n4pDh30LdyOOwhe0qyW"
]
}
GET /datasets/{DATASET_ID}/classes/{CLASS_ID}/clusters/{CLUSTER_NAME}/images/
Retrieve a list of Image objects by a cluster name (all images that belongs to that cluster).
Returns: Pagination object with list of Image objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{class_id}/clusters/cluster_main/images/
# coming soon
Example response:
{
"total": 2,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/datasets/9538e44c-6f30-40b7-8d7c-73bda1d41a9e/class/3d08d7c2-6e15-4a48-9cbd-c04dc257b4f8/clusters/cluster_main/images/?limit=5&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/9538e44c-6f30-40b7-8d7c-73bda1d41a9e/class/3d08d7c2-6e15-4a48-9cbd-c04dc257b4f8/clusters/cluster_main/images/?limit=5&offset=0",
"data": [
{
"id": "0a98ca4b-6c88-42e8-99d6-24d273f21e23",
"file_uri": "storage://V4Ic75h6RYqKXK9TkkDw",
"time_created": "2020-01-01 00:00:00.786000",
"time_updated": "2020-01-01 00:00:00.786000",
"active": true,
"notes": "This is a text",
"is_auto_generated": false,
"evaluation_result": {
"face_attributes": {
"emotion": "Calm",
"smile": false,
"eyeglasses": false,
"sunglasses": false,
"eyesopen": true,
"mouthopen": false,
"beard": false,
"mustache": false,
"roll": -15,
"yaw": 35.97,
"pitch": -8.71
},
"face_clustering": "cluster_main"
}
},
{
"id": "1198ca4b-6c88-42e8-99d6-24d273f21e31",
"file_uri": "storage://t7r2deRbEW8SxZy3oHyX",
"time_created": "2020-01-01 00:00:00.786000",
"time_updated": "2020-01-01 00:00:00.786000",
"active": true,
"notes": "",
"is_auto_generated": false,
"evaluation_result": {
"face_attributes": {
"emotion": "Calm",
"smile": false,
"eyeglasses": false,
"sunglasses": false,
"eyesopen": true,
"mouthopen": false,
"beard": false,
"mustache": false,
"roll": 30,
"yaw": 25.30,
"pitch": 2.31
},
"face_clustering": "cluster_main"
}
}
]
}
/Images
GET /datasets/{DATASET_ID}/classes/{CLASS_ID}/images/
Retrieve a list of Image objects.
Returns: Pagination object with list of Image objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{image_id}/images/
# coming soon
Example response:
{
"total": 1,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/datasets/9006d642-db4c-48fd-8869-5f5a5f8e1419/classes/c47cc3ba-a49f-4304-b331-58444d306d58/images/?limit=10&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/9006d642-db4c-48fd-8869-5f5a5f8e1419/classes/c47cc3ba-a49f-4304-b331-58444d306d58/images/?limit=10&offset=0",
"data": [
{
"id": "12c5d48f-3a4a-45cc-959d-a19566ca4773",
"file_uri": "storage://6fJYINhjbPBhvpuyCXxZ",
"time_created": "2020-08-26 08:32:41.535000",
"time_updated": "2020-08-26 08:32:41.535000",
"active": true,
"notes": "",
"is_auto_generated": false,
"evaluation_result": {}
}
]
}
GET /datasets/{DATASET_ID}/classes/{CLASS_ID}/images/{IMAGE_ID}/
Retrieves a single Image object by its ID
Returns: Image object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{class_id}/images/{image_id}/
# coming soon
Example response:
{
"total": 1,
"offset": 0,
"limit": 10,
"next": "http://api.deepva.com/api/v1/datasets/9006d642-db4c-48fd-8869-5f5a5f8e1419/classes/c47cc3ba-a49f-4304-b331-58444d306d58/images/?limit=10&offset=10",
"prev": "http://api.deepva.com/api/v1/datasets/9006d642-db4c-48fd-8869-5f5a5f8e1419/classes/c47cc3ba-a49f-4304-b331-58444d306d58/images/?limit=10&offset=0",
"data": [
{
"id": "12c5d48f-3a4a-45cc-959d-a19566ca4773",
"file_uri": "storage://6fJYINhjbPBhvpuyCXxZ",
"time_created": "2020-08-26 08:32:41.535000",
"time_updated": "2020-08-26 08:32:41.535000",
"active": true,
"notes": "",
"is_auto_generated": false,
"evaluation_result": {}
}
]
}
POST /datasets/{DATASET_ID}/classes/{CLASS_ID}/images/
Creates a new Image
Returns: Image object that has been created
Example request:
curl -d '{"file_uri": "storage://6fJYINhjbPBhvpuyCXxZ"}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/datasets/{dataset_id}/classes/{class_id}/images/
# coming soon
Example response:
{
"id": "12c5d48f-3a4a-45cc-959d-a19566ca4773",
"file_uri": "storage://6fJYINhjbPBhvpuyCXxZ",
"time_created": "2020-08-26 08:32:41.535313",
"time_updated": "2020-08-26 08:32:41.535322",
"active": true,
"notes": "",
"is_auto_generated": false,
"evaluation_result": {}
}
DELETE /datasets/{DATASET_ID}/classes/{CLASS_ID}/images/{IMAGE_ID}/
Deletes an Image
Returns: Message saying that the image has been deleted
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v1/datasets/{DATASET_ID}/classes/{CLASS_ID}/{CLASS_ID}/images/{IMAGE_ID}/
# coming soon
Example response:
{
"message": "Image has been deleted"
}
Custom Training and Models
/Trainings
POST /trainings/
Creates a new Training
Returns: Training object that has been created
Example request:
curl -d '{ "source_dataset_id": "b97e20c8-3e68-4831-8eaf-e7a592d3a802", "output_model_name": "My Model", "output_model_description": "This is a model which training was started via Postman", "output_model_changelog": "initial training", "config": {} }' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/trainings/
# coming soon
Example response:
{
"id": "174f8cd7-64a1-4b0c-8735-cbbc80d24024",
"type": "face",
"state": "waiting",
"progress": 0,
"duration": 0,
"error": "",
"time_created": "2020-12-15 11:39:22.792673",
"time_started": null,
"time_completed": null,
"source_dataset": {
"id": "b97e20c8-3e68-4831-8eaf-e7a592d3a802",
"name": "Test",
"description": "A test dataset",
"version": 1.0,
"type": "face",
"time_created": "2020-10-30 17:34:41.480000",
"time_updated": "2020-10-30 17:34:41.480000",
"number_of_classes": 0,
"number_of_active_classes": 0,
"number_of_images": 0,
"number_of_active_images": 0,
"preview_images": [],
"evaluation_result": {
"gender_statistics": {
"male": 5
}
},
"evaluation_state": 0,
"issues": {
"has_error": true,
"has_warning": false
}
},
"output_model_name": "My Model",
"output_model": null,
"config": {},
"output_model_description": "This is a model which training was started via Postman",
"output_model_changelog": "initial training"
}
The Training has just been created. The state
is "waiting" becasue it waits for processing by DeepVA. As soon as the job is done (state = "completed") you will see your new custom trained model at https://api.deepva.com/api/v1/models/
(models endpoint).
You can request the Training by its ID and check the state: https://api.deepva.com/v1/trainings/174f8cd7-64a1-4b0c-8735-cbbc80d24024/
/Models
TODO
Storage
If you can not provide the URL of your images or videos that you want to send to the API, you can use our /storage endpoint. You can upload your media file and specify the storage URI (storage://q1gyaldQ146pAagevHIK) as source when creating a job.
GET /storage?folder={FOLDER_PATH}
Retrieve a list of storage objects
Returns: Pagination object with list of Storage objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
folder
- Path of the folder
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {api-key}" "https://api.deepva.com/api/v2/storage?folder={folder_path}"
API_KEY = "{api-key}"
response = requests.get("https://api.deepva.com/api/v2/storage/",
data={'folder': "/"},
headers={'Authorization': 'Key {0}'.format(API_KEY)}
)
if response.status_code == 200:
storage_list = response.json()
print(storage_list)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 3,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"id": 123579,
"url": "storage://SzSVeq9FWjgiwMUL2e85",
"file_size": 6966,
"name": "mm",
"uploaded": true,
"upload_failed": false,
"storage_type": "IMAGE",
"uploaded_at": "2023-03-10T18:14:56.623460Z"
},
{
"id": 123580,
"url": "storage://Op6tM7vlQMNbOgogOHkU",
"file_size": 7540,
"name": "mm2",
"uploaded": true,
"upload_failed": false,
"storage_type": "IMAGE",
"uploaded_at": "2023-03-10T18:14:56.779741Z"
},
{
"id": 123575,
"url": null,
"file_size": null,
"name": "/Disney - Images[ae5b8152]/",
"uploaded": true,
"upload_failed": false,
"storage_type": "FOLDER",
"uploaded_at": "2023-03-10T18:14:56.598659Z"
}
]
}
GET /storage/{STORAGE_ID}/
Retrieve a storage object
Returns: Storage object
Example request:
curl -v -H "Authorization: Key {api-key}" https://api.deepva.com/api/v2/storage/{storage_id}
API_KEY = "{api-key}"
STORAGE_ID = "{storage_id}"
response = requests.get(f"https://api.deepva.com/api/v2/storage/{STORAGE_ID}",
headers={f"'Authorization': 'Key {API_KEY}'"}
)
if response.status_code == 200:
storage = response.json()
print(storage)
else:
print(f"Error: {response.status_code}")
Example response:
{
"id": 123579,
"url": "storage://SzSVeq9FWjgiwMUL2e85",
"file_size": 6966,
"name": "mm",
"uploaded": true,
"upload_failed": false,
"storage_type": "IMAGE",
"uploaded_at": "2023-03-10T18:14:56.623460Z"
}
GET /storage/{STORAGE_ID}/file/
Download the storage file
Returns: Corresponding storage file
Example request:
curl -v -H "Authorization: Key {api-key}" https://api.deepva.com/api/v2/storage/{storage_id}/file
API_KEY = "{api-key}"
STORAGE_ID = "{storage_id}"
response = requests.get(f"https://api.deepva.com/api/v2/storage/{STORAGE_ID}/file",
headers={f"'Authorization': 'Key {API_KEY}'"}
)
if response.status_code == 200:
storage_file = response.content
print(storage_file)
else:
print(f"Error: {response.status_code}")
GET /storage/{STORAGE_ID}/thumbnail/
Download the thumbnail of the storage file
Returns: Corresponding storage thumbnail file
Example request:
curl -v -H "Authorization: Key {api-key}" https://api.deepva.com/api/v2/storage/{storage_id}/thumbnail
API_KEY = "{api-key}"
STORAGE_ID = "{storage_id}"
response = requests.get(f"https://api.deepva.com/api/v2/storage/{STORAGE_ID}/thumbnail",
headers={f"'Authorization': 'Key {API_KEY}'"}
)
if response.status_code == 200:
thumbnail = response.content
print(thumbnail)
else:
print(f"Error: {response.status_code}")
POST /storage/
Upload a file to the storage
Returns: Storage object that has been created
Note: A
folder
parameter with a path is required when uploading a file. If the specified folder does not exist, it is automatically created.
Example request:
curl -H "Authorization: Key {api-key}" -F "folder=/" -F "file=@{path-to-file}" https://api.deepva.com/api/v2/storage/
API_KEY = "{api-key}"
multipart_form_data = {
'file': (os.path.basename(file), open("example.jpg", 'rb'))
}
response = requests.post("https://api.deepva.com/api/v2/storage/",
files=multipart_form_data,
data={'folder': "/"},
headers={f"'Authorization': 'Key {API_KEY}'"}
)
if response.status_code == 201:
return response.json()['url'] # storage url (e.g. storage://uo7hRJfapCQKZnhXGl78)
else:
raise RuntimeError("Upload failed!")
var apiKey = "Key xyz"; // DeepVA API key e.g. "Key xyz"
var folderName = "/"; // Destination folder (e.g. "/my-subfolder/" or "/" for root folder)
var imageFileName = "example.jpg"; // Remote filename
FileStream fs = File.Open("path-to-local-file/example.jpg", FileMode.Open, FileAccess.Read, FileShare.None); // File to upload
HttpClient httpClient = new HttpClient();
httpClient.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
httpClient.DefaultRequestHeaders.Add("Authorization", apiKey);
MultipartFormDataContent multiForm = new MultipartFormDataContent();
multiForm.Add(new StringContent(folderName), "folder");
multiForm.Add(new StreamContent(fs), "file", imageFileName);
HttpResponseMessage response = await httpClient.PostAsync("https://api.deepva.com/api/v2/storage/", multiForm);
response.EnsureSuccessStatusCode();
httpClient.Dispose();
Example response:
{
"id": 123579,
"url": "storage://SzSVeq9FWjgiwMUL2e85",
"file_size": 6966,
"name": "mm",
"uploaded": true,
"upload_failed": false,
"storage_type": "IMAGE",
"uploaded_at": "2023-03-10T18:14:56.623460Z"
}
DELETE /storage/{STORAGE_ID}/
Deletes a storage and the contents within the storage
Returns: 200 OK status code
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v2/storage/{storage_id}/
API_KEY = "{api-key}"
STORAGE_ID = "{storage_id}"
response = requests.delete(f"https://api.deepva.com/api/v2/storage/{STORAGE_ID}",
headers={f"'Authorization': 'Key {API_KEY}'"}
)
if response.status_code == 200:
print("Storage deleted!")
else:
raise RuntimeError("Storage deletion failed!")
Dictionaries
GET /dictionaries/
Retrieve a list of dictionary objects
Returns: Pagination object with list of Dictionary objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination and Filter for more information.
Example request:
curl -v -H "Authorization: Key {api-key}" "https://api.deepva.com/api/v2/dictionaries"
API_KEY = "{api-key}"
response = requests.get(
"https://api.deepva.com/api/v2/dictionaries/",
headers={
"Authorization": f"Key {API_KEY}"
}
)
if response.status_code == 200:
dictionary_list = response.json()
print(dictionary_list)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 3,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"id": "672bcee8-605d-4b53-9874-ce9c12f47ca8",
"name": "A",
"type": "map",
"language": "german",
"time_created": "2023-06-02T10:19:46.020876Z",
"is_public": false
},
{
"id": "9c6ec3b5-1cd0-462c-821a-d47d5a770b4d",
"name": "B",
"type": "simple",
"language": "english",
"time_created": "2023-05-10T08:59:15.182682Z",
"is_public": false
},
{
"id": "927c27b8-b2a2-41d8-b9f7-dce33e6f65a3",
"name": "C",
"type": "simple",
"language": "croatian",
"time_created": "2023-05-03T13:06:51.499598Z",
"is_public": false
}
]
}
GET /dictionaries/{DICTIONARY_ID}/
Retrieve a dictionary object
Returns: Dictionary object
Example request:
curl -v -H "Authorization: Key {api-key}" https://api.deepva.com/api/v2/dictionaries/{dictionary_id}
API_KEY = "{api-key}"
DICTIONARY_ID = "{dictionary_id}"
response = requests.get(
f"https://api.deepva.com/api/v2/dictionaries/{DICTIONARY_ID}",
headers={
"Authorization": f"Key {API_KEY}"
}
)
if response.status_code == 200:
dictionary = response.json()
print(dictionary)
else:
print(f"Error: {response.status_code}")
Example response:
{
"id": "672bcee8-605d-4b53-9874-ce9c12f47ca8",
"name": "A",
"type": "map",
"language": "german",
"time_created": "2023-06-02T10:19:46.020876Z",
"is_public": false
}
GET /dictionaries/{DICTIONARY_ID}/file
Retrieve a dictionary file
Returns: Dictionary file
Example request:
curl -v -H "Authorization: Key {api-key}" https://api.deepva.com/api/v2/dictionaries/{dictionary_id}/file
API_KEY = "{api-key}"
DICTIONARY_ID = "{dictionary_id}"
response = requests.get(
f"https://api.deepva.com/api/v2/dictionaries/{DICTIONARY_ID}/file",
headers={
"Authorization": f"Key {API_KEY}"
}
)
if response.status_code == 200:
dictionary_file = response.content
print(dictionary_file)
else:
print(f"Error: {response.status_code}")
POST /dictionaries/
Upload a text file as a dictionary
Returns: Dictionary object that has been created
Form Data Parameters:
name
- Name of the dictionary
file
- File representing the dictionary. For the content type and its corresponding format, see here.
language
- Language of the dictionary. For more information, see here. Optional.
Example request:
curl -H "Authorization: Key {api-key}" -F "name={dictionary-name}" -F "file=@{path-to-file};type={file-content-type}" -F "language={dictionary-language}" https://api.deepva.com/api/v2/dictionaries/
API_KEY = "{api-key}"
multipart_form_data = {
"file": (os.path.basename(file), open("dictionary.txt", "rb"), "text/plain")
}
response = requests.post(
"https://api.deepva.com/api/v2/dictionaries/",
files=multipart_form_data,
data={
"name": "City names",
"language": "any",
},
headers={
"Authorization": f"Key {API_KEY}"
}
)
if response.status_code == 201:
return response.json()
else:
raise RuntimeError("Upload failed!")
Example response:
{
"id": "672bcee8-605d-4b53-9874-ce9c12f47ca8",
"name": "City names",
"type": "simple",
"language": "any",
"time_created": "2023-06-02T10:19:46.020876Z",
"is_public": false
}
DELETE /dictionaries/{STORAGE_ID}/
Deletes a dictionary
Returns: 200 OK status code
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v2/dictionaries/{dictionary_id}/
API_KEY = "{api-key}"
DICTIONARY_ID = "{dictionary_id}"
response = requests.delete(
f"https://api.deepva.com/api/v2/dictionaries/{DICTIONARY_ID}",
headers={
"Authorization": f"Key {API_KEY}"
}
)
if response.status_code == 200:
print("Dictionary deleted!")
else:
raise RuntimeError("Dictionary deletion failed!")
Knowledge Graph
GET /knowledge-graph/nodes/
Retrieve a list of nodes in the graph
Returns: Pagination object with a list of Node Summary objects
URL Parameters:
type
- Only list nodes of this type (Available: person
, asset
, subasset
, broadcast
). Optional.
search
- Query string used to perform case-insensitive substring search across nodes' static attributes. Optional.
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0). Optional.
limit
- A limit on the number of objects to be returned (default: 256, max: 256). Optional.
This endpoint also supports Attribute-based Filtering.
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/nodes/?type=person&limit=5"
# coming soon
Example response:
{
"total": 10475,
"next": "https://api.deepva.com/api/v1/knowledge-graph/nodes/?limit=5&offset=5&type=person",
"prev": null,
"limit": 5,
"offset": 0,
"data": [
{
"id": 18378,
"custom_id": "Q22686",
"name": "Donald Trump",
"type": "person",
"relevance": {
"type": "pagerank",
"value": 76.732
}
},
{
"id": 16227,
"custom_id": "Q567",
"name": "Angela Merkel",
"type": "person",
"relevance": {
"type": "pagerank",
"value": 58.0493
}
},
{
"id": 17463,
"custom_id": "Q6279",
"name": "Joe Biden",
"type": "person",
"relevance": {
"type": "pagerank",
"value": 28.6642
}
},
{
"id": 31979,
"custom_id": "Q86294",
"name": "Jens Spahn",
"type": "person",
"relevance": {
"type": "pagerank",
"value": 27.7049
}
},
{
"id": 17299,
"custom_id": "Q78205",
"name": "Armin Laschet",
"type": "person",
"relevance": {
"type": "pagerank",
"value": 27.0948
}
}
]
}
GET /knowledge-graph/nodes/{NODE_ID}/
Retrieve static attributes of the node with an ID of {NODE_ID}
Returns: Node Description object
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/nodes/24478/"
# coming soon
Example response:
{
"id": 24478,
"custom_id": "Q44068",
"name": "Dirk Nowitzki",
"description": "German professional basketball player",
"type": "person"
}
GET /knowledge-graph/nodes/{NODE_ID}/attributes/
Retrieve all attributes of the node with an ID of {NODE_ID}
Returns: Pagination object with a list of Attribute objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0). Optional.
limit
- A limit on the number of objects to be returned (default: 256, max: 256). Optional.
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/nodes/24478/attributes/"
# coming soon
Example response:
{
"total": 54,
"next": null,
"prev": null,
"limit": 256,
"offset": 0,
"data": [
{
"name": "date_of_birth",
"value": "19.06.1978."
},
{
"name": "custom_id",
"value": "Q44068"
},
{
"name": "name",
"value": "Dirk Nowitzki"
},
{
"name": "description",
"value": "German professional basketball player"
},
...
]
GET /knowledge-graph/nodes/{NODE_ID}/similar/
Retrieve a list of nodes similar to the node with an ID of {NODE_ID}
Returns: Pagination object with a list of Node Summary objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0). Optional.
limit
- A limit on the number of objects to be returned (default: 256, max: 256). Optional.
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/nodes/24478/similar/?limit=2"
# coming soon
Example response:
{
"total": 4115,
"next": "http://testenv.deepva.com/api/v1/knowledge-graph/nodes/24478/similar?limit=2&offset=2",
"prev": null,
"limit": 2,
"offset": 0,
"data": [
{
"id": 36209,
"custom_id": "Q36159",
"name": "LeBron James",
"type": "person",
"relevance": {
"type": "similar",
"value": 17
}
},
{
"id": 27836,
"custom_id": "Q25369",
"name": "Kobe Bryant",
"type": "person",
"relevance": {
"type": "similar",
"value": 15
}
}
]
}
GET /knowledge-graph/nodes/{NODE_ID}/related/
Retrieve a list of nodes related to the node with an ID of {NODE_ID}
Returns: Pagination object with a list of Node Summary objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0). Optional.
limit
- A limit on the number of objects to be returned (default: 256, max: 256). Optional.
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/nodes/24478/related/?limit=2"
# coming soon
Example response:
{
"total": 52,
"next": "http://testenv.deepva.com/api/v1/knowledge-graph/nodes/24478/related?limit=2&offset=2",
"prev": null,
"limit": 2,
"offset": 0,
"data": [
{
"id": 13103,
"custom_id": "Q183",
"name": "Germany",
"type": "node",
"relevance": {
"type": "related",
"value": 2
}
},
{
"id": 15922,
"custom_id": "Q2999",
"name": "Würzburg",
"type": "node",
"relevance": {
"type": "related",
"value": 2
}
}
]
}
GET /knowledge-graph/nodes/{NODE_ID}/attributes/compare/{OTHER_NODE_ID}/
Compare attributes of the (reference) node with an ID of {NODE_ID}
against the attributes of the (query) node with an ID of {OTHER_NODE_ID}
Returns: Pagination object with a list of Attribute Comparison objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0). Optional.
limit
- A limit on the number of objects to be returned (default: 256, max: 256). Optional.
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/nodes/24478/attributes/compare/36209/"
# coming soon
Example response:
{
"total": 54,
"next": null,
"prev": null,
"limit": 256,
"offset": 0,
"data": [
{
"name": "place_of_birth",
"value": "Würzburg",
"common": false
},
{
"name": "occupation",
"value": "basketball player",
"common": true
},
...
]
}
GET /knowledge-graph/attribute-descriptions/
Retrieve a list of available attributes in the graph
Returns: Pagination object with list of Attribute Description objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0). Optional.
limit
- A limit on the number of objects to be returned (default: 256, max: 256). Optional.
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/attribute-descriptions/?limit=5"
# coming soon
Example response:
{
"total": 131580,
"next": "https://api.deepva.com/api/v1/knowledge-graph/attribute-descriptions/?limit=5&offset=5",
"prev": null,
"limit": 5,
"offset": 0,
"data": [
{
"name": "name",
"description": null, # Coming soon
"static": true,
"type": "str"
},
{
"name": "date",
"description": null,
"static": true,
"type": "date"
},
{
"name": "height",
"description": null,
"static": true,
"type": "float"
},
{
"name": "occupation",
"description": null,
"static": false,
"type": "str"
},
{
"name": "sex_or_gender",
"description": null,
"static": false,
"type": "str"
}
]
}
GET /knowledge-graph/search-suggestions
Get top 5 search suggestions based on the query string
Returns: A list of search suggestion strings
URL Parameters:
query
- Query string used for suggesting search terms
attribute
- The node attribute for suggesting search terms (e.g. place_of_birth)
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/search-suggestions/?attribute=place_of_birth&query=frei"
# coming soon
Example response:
[
"Freiburg im Breisgau",
"Freie Universität Berlin",
"Freie Wähler",
"Freitag",
"Freida Parton"
]
POST /knowledge-graph/import/{FORMAT}
Import data to the knowledge graph, in one of the supported formats
Returns: Import Status object
Example request:
curl -d '{"apply_ner": true, "broadcasts": [{"id": "123", "date": "2000-01-01", "asset_id": null, "title": "Broadcast #101", "description": null, "show": null, "people": [{"full_name": "Angela Merkel"}]}]}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST "https://api.deepva.com/api/v1/knowledge-graph/import/broadcast"
# coming soon
Example response:
{
"id": "f1386be2-0fb6-4775-b125-126562481030",
"time_created": "2022-04-19T15:15:18.634500Z",
"time_completed": null,
"state": "waiting"
}
GET /knowledge-graph/import/{FORMAT}/{IMPORT_ID}
Check the current status of the import with an ID of IMPORT_ID
Returns: Import Status object
Example request:
curl -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" "https://api.deepva.com/api/v1/knowledge-graph/import/broadcast/f1386be2-0fb6-4775-b125-126562481030"
# coming soon
Example response:
{
"id": "f1386be2-0fb6-4775-b125-126562481030",
"time_created": "2022-04-19T15:15:18.634500Z",
"time_completed": "2022-04-19T15:20:01.634500Z",
"state": "completed"
}
Batches & Reports
/Batches
GET /batches/
Retrieve a list of all batches
Returns: Pagination object with list of Batch objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/batches/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/batches/",
headers=headers)
if response.status_code == 200:
batches = response.json()
print(batches)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 3,
"offset": 0,
"limit": 10,
"next": null,
"prev": null,
"data": [
{
"id": "46c1d2ff-8e1c-4843-9b6d-71290dd75ec7",
"name": "batch_report_split_file1",
"description": "",
"parent": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"time_created": "2023-03-09 12:51:05.644000",
"time_updated": "2023-03-09 12:51:05.644000"
},
{
"id": "bb6e8a36-4b94-4dda-9428-746c2e91bc3a",
"name": "batch_report_split_file2",
"description": "",
"parent": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"time_created": "2023-03-09 12:50:31.388000",
"time_updated": "2023-03-09 12:50:31.388000"
},
{
"id": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"name": "top-level",
"description": "",
"parent": null,
"time_created": "2023-03-09 12:47:20.890000",
"time_updated": "2023-03-09 12:47:20.890000"
}
]
}
GET /batches/{BATCH_ID}/
Retrieves a single batch by its ID
Returns: Batch object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/batches/{batch_id}/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/batches/{batch_id}/",
headers=headers)
if response.status_code == 200:
batch = response.json()
print(batch)
else:
print(f"Error: {response.status_code}")
Example response:
{
"id": "46c1d2ff-8e1c-4843-9b6d-71290dd75ec7",
"name": "batch_report_split_file1",
"description": "",
"parent": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"time_created": "2023-03-09 12:51:05.644000",
"time_updated": "2023-03-09 12:51:05.644000"
}
GET /batches/{BATCH_ID}/sub-batches/
Retrieve a list of all sub-batches within a batch
Returns: Pagination object with list of Batch objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/batches/{batch_id}/sub-batches/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/batches/{batch_id}/sub-batches/",
headers=headers)
if response.status_code == 200:
batches = response.json()
print(batches)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 2,
"offset": 0,
"limit": 10,
"next": null,
"prev": null,
"data": [
{
"id": "46c1d2ff-8e1c-4843-9b6d-71290dd75ec7",
"name": "batch_report_split_file1",
"description": "",
"parent": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"time_created": "2023-03-09 12:51:05.644000",
"time_updated": "2023-03-09 12:51:05.644000"
},
{
"id": "bb6e8a36-4b94-4dda-9428-746c2e91bc3a",
"name": "batch_report_split_file2",
"description": "",
"parent": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"time_created": "2023-03-09 12:50:31.388000",
"time_updated": "2023-03-09 12:50:31.388000"
}
]
}
GET /batches/{BATCH_ID}/jobs/
Retrieve a list of all mining jobs belonging to the batch
Returns: Pagination object with list of Job objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/batches/{batch_id}/jobs/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/batches/{batch_id}/jobs/",
headers=headers)
if response.status_code == 200:
jobs = response.json()
print(jobs)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 2,
"offset": 0,
"limit": 10,
"next": null,
"prev": null,
"data": [
{
"id": "d990850d-435f-44e9-bc6d-bb2619750c55",
"tag": "",
"state": "completed",
"errors": [],
"progress": 1,
"duration": 17.195,
"time_created": "2023-03-09 12:50:31.419000",
"time_started": "2023-03-09 12:50:31.500000",
"time_completed": "2023-03-09 12:50:48.695000",
"sources": [
"https://demo.deepva.com/test-files/4.mp4"
],
"modules": {
"advanced_diversity_analysis": {
"state": "completed",
"progress": 1
}
},
"media_type": "video",
"is_synced_to_kg": false,
"result": {
"detailed_link": "https://api.deepva.com/v1/jobs/d990850d-435f-44e9-bc6d-bb2619750c55/detailed-results/",
"summary": [
{
"source": "https://demo.deepva.com/test-files/4.mp4",
"media_type": "video",
"info": {
"fps": 50.0,
"resolution": [
1280,
720
],
"total_frames": 422,
"duration": 8.44
},
"items": []
}
]
}
},
{
"id": "61d8c7cf-569c-4fc7-816a-0ef97d598741",
"tag": "",
"state": "completed",
"errors": [],
"progress": 1,
"duration": 24.595,
"time_created": "2023-03-09 12:50:31.453000",
"time_started": "2023-03-09 12:50:49.800000",
"time_completed": "2023-03-09 12:51:14.395000",
"sources": [
"https://demo.deepva.com/test-files/5.mp4"
],
"modules": {
"advanced_diversity_analysis": {
"state": "completed",
"progress": 1
}
},
"media_type": "video",
"is_synced_to_kg": false,
"result": {
"detailed_link": "https://api.deepva.com/v1/jobs/61d8c7cf-569c-4fc7-816a-0ef97d598741/detailed-results/",
"summary": [
{
"source": "https://demo.deepva.com/test-files/5.mp4",
"media_type": "video",
"info": {
"fps": 60.0,
"resolution": [
1280,
720
],
"total_frames": 805,
"duration": 13.416666666666666
},
"items": []
}
]
}
}
]
}
POST /batches/
Creates a new batch
Returns: Batch object that has been created
Example request:
curl -d '{"name": "top-level"}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/batches/
# coming soon
Example response:
{
"id": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"name": "top-level",
"description": "",
"parent": null,
"time_created": "2023-03-09 12:47:20.890337",
"time_updated": "2023-03-09 12:47:20.890349"
}
POST /batches/files/
Creates a new batch from source files (e.g, "CSV")
Returns: A List with information on the processing of the files and associated Batch object that has been created
Form fields:
files
- A list of files
parent_id
- Parent batch ID for a batch to be created created from source files. Optional.
parent_name
- Name of parent batch to be created, for which the created batch from files becomes the sub-batch. Optional.
Warning The endpoint can have either parent_id or parent_name but not both in the form fields
Example request:
curl -H "Authorization: Key {your-api-key}" -X POST -F 'files=@{path-to-file}' -F 'parent_id={parent_id}' https://api.deepva.com/api/v1/batches/
# coming soon
Example response:
[
{
"file": "batch_report_split_file1.csv",
"success": true,
"error": null,
"batch": {
"id": "46c1d2ff-8e1c-4843-9b6d-71290dd75ec7",
"name": "batch_report_split_file1",
"description": "",
"parent": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"time_created": "2023-03-09 12:51:05.644959",
"time_updated": "2023-03-09 12:51:05.644968"
}
}
]
POST /batches/{BATCH_ID}/report/
Creates a new report on a batch
Returns: Report object that has been created
Example request:
curl -H "Authorization: Key {your-api-key}" -X POST https://api.deepva.com/api/v1/batches/{batch_id}/report/
# coming soon
Example response:
{
"id": "8d3e24ce-a422-4de2-bcf2-2fc135ac1abf",
"title": "Report of top-level on 09.03.2023",
"time_created": "2023-03-09T12:54:49.076399Z",
"type": "diversity",
"state": "waiting",
"error_msg": null
}
DELETE /batches/{BATCH_ID}/
Deletes a batch and its sub-batches and aborts all its mining jobs
Returns: Message saying that a batch or batches has been deleted
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v1/batches/{batch_id}/
# coming soon
Example response:
{
"message": "Batch or batches has been deleted"
}
/Reports
GET /reports/
Retrieve a list of all Reports
Returns: Pagination object with list of Report objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/reports/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/reports/",
headers=headers)
if response.status_code == 200:
reports = response.json()
print(reports)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 3,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"id": "fddae0a5-94d9-4d44-bd2a-2eccb066b79d",
"title": "Report of batch_report_file on 01.03.2023",
"time_created": "2023-03-01T12:58:51.788296Z",
"type": "diversity",
"state": "completed",
"error_msg": null
},
{
"id": "ab65d9a9-3216-49ba-88c7-44dba4558339",
"title": "Report of batch_report_file2 on 09.03.2023",
"time_created": "2023-03-09T11:05:28.787840Z",
"type": "diversity",
"state": "completed",
"error_msg": null
},
{
"id": "8d3e24ce-a422-4de2-bcf2-2fc135ac1abf",
"title": "Report of top-level on 09.03.2023",
"time_created": "2023-03-09T12:54:49.076399Z",
"type": "diversity",
"state": "completed",
"error_msg": null
}
]
}
GET /reports/{REPORT_ID}/
Retrieves a single Report by its ID
Returns: Report object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/reports/{report_id}/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/reports/{report_id}/",
headers=headers)
if response.status_code == 200:
report = response.json()
print(report)
else:
print(f"Error: {response.status_code}")
Example response:
{
"id": "fddae0a5-94d9-4d44-bd2a-2eccb066b79d",
"title": "Report of batch_report_file on 01.03.2023",
"time_created": "2023-03-01T12:58:51.788296Z",
"type": "diversity",
"state": "completed",
"error_msg": null
}
GET /reports/{REPORT_ID}/items/
Retrieve a list of all report items
Returns: Pagination object with list of ReportItem objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/reports/{report_id}/items/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/reports/{report_id}/items/",
headers=headers)
if response.status_code == 200:
report_items = response.json()
print(report_items)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 3,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"content": {
"batch_id": "46c1d2ff-8e1c-4843-9b6d-71290dd75ec7",
"report_info": {
"gender_count": {
"female": 1
},
"age_count": {
"20-30": 1
},
"person_screen_time": {
"female_1": 8.64
},
"gender_screen_time": {
"female": 8.64
},
"age_screen_time": {
"20-30": 8.64
},
"gender_speech_time": {
"female": 8.64
},
"age_speech_time": {
"20-30": 8.64
},
"name_to_speech_time": {
"female_1": 8.64
}
}
}
},
{
"content": {
"batch_id": "bb6e8a36-4b94-4dda-9428-746c2e91bc3a",
"report_info": {
"gender_count": {
"male": 4,
"female": 2
},
"age_count": {
"30-40": 5,
"20-30": 1
},
"person_screen_time": {
"male_1": 14.0,
"male_2": 19.0,
"female_1": 7.0,
"female_2": 3.5
},
"gender_screen_time": {
"male": 33.0,
"female": 10.5
},
"age_screen_time": {
"30-40": 36.5,
"20-30": 7.0
},
"gender_speech_time": {
"male": 17.0,
"female": 3.5
},
"age_speech_time": {
"30-40": 20.5,
"20-30": 0
},
"name_to_speech_time": {
"male_1": 0,
"male_2": 17.0,
"female_1": 0,
"female_2": 3.5
}
}
}
},
{
"content": {
"batch_id": "c47859ee-a39d-45ed-ad54-8e6ba060be80",
"report_info": {
"gender_count": {
"female": 3,
"male": 4
},
"age_count": {
"20-30": 2,
"30-40": 5
},
"person_screen_time": {
"female_1": 15.64,
"male_1": 14.0,
"male_2": 19.0,
"female_2": 3.5
},
"gender_screen_time": {
"female": 19.14,
"male": 33.0
},
"age_screen_time": {
"20-30": 15.64,
"30-40": 36.5
},
"gender_speech_time": {
"female": 12.14,
"male": 17.0
},
"age_speech_time": {
"20-30": 8.64,
"30-40": 20.5
},
"name_to_speech_time": {
"female_1": 8.64,
"male_1": 0,
"male_2": 17.0,
"female_2": 3.5
}
}
}
}
]
}
DELETE /reports/{REPORT_ID}/
Deletes the report
Returns: 204 No Content
Example request:
curl -H "Authorization: Key {your-api-key}" -X DELETE https://api.deepva.com/api/v1/reports/{report_id}/
# coming soon
Exports
GET /exports/
Retrieve a list of all exports
Returns: Pagination object with list of Export objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/exports/
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/exports/",
headers=headers)
if response.status_code == 200:
exports = response.json()
print(exports)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 2,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"id": "ce659a6a-a684-4e91-81d0-aaab50d1bd06",
"strategy": "basic_dataset_export",
"time_created": "2023-03-10T18:15:32.358354Z",
"time_completed": null,
"state": "completed",
"progress": 1.0,
"error_msg": null,
"config": {
"dataset_id": "ae5b8152-e60f-4918-a59e-20e6d875914c"
}
},
{
"id": "26cb5642-bf1e-4ec2-895a-445b19b1f168",
"strategy": "iais_audio_dataset_export",
"time_created": "2023-03-15T14:10:27.566567Z",
"time_completed": "2023-03-15T14:10:28.566567Z",
"state": "completed",
"progress": 0.0,
"error_msg": null,
"config": {
"dataset_id": "ae5b8152-e60f-4918-a59e-20e6d875914c"
}
}
]
}
GET /exports/{EXPORT_ID}/
Retrieve an export object by its ID
Returns: Export object
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/exports/{export_id}
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/exports/{export_id}",
headers=headers)
if response.status_code == 200:
export = response.json()
print(export)
else:
print(f"Error: {response.status_code}")
Example response:
{
"id": "26cb5642-bf1e-4ec2-895a-445b19b1f168",
"strategy": "basic_dataset_export",
"time_created": "2023-03-15T14:10:27.566567Z",
"time_completed": null,
"state": "completed",
"progress": 1.0,
"error_msg": null,
"config": {
"dataset_id": "ae5b8152-e60f-4918-a59e-20e6d875914c"
}
}
POST /exports/
Trigger an export and create the corresponding export object
Returns: Export object
Example request:
curl -d '{"strategy": {export_strategy}, "config": {"dataset_id": {dataset_id}}}' -H "Content-Type: application/json" -H "Authorization: Key {your-api-key}" -X POST "https://api.deepva.com/api/v1/exports"
# coming soon
Example response:
{
"id": "26cb5642-bf1e-4ec2-895a-445b19b1f168",
"strategy": "basic_dataset_export",
"time_created": "2023-03-15T14:10:27.566567Z",
"time_completed": null,
"state": "waiting",
"progress": 0.0,
"error_msg": null,
"config": {
"dataset_id": "ae5b8152-e60f-4918-a59e-20e6d875914c"
}
}
GET /exports/{EXPORT_ID}/artifacts/
Retrieve a list of all artifacts of an export
Returns: Pagination object with list of Artifact objects
URL Parameters:
offset
- The index of the first object in the list to be returned, starting with 0 (default: 0)
limit
- A limit on the number of objects to be returned (default: 10)
See Pagination for more information.
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/exports/{export_id}/artifacts
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/exports/{export_id}/artifacts",
headers=headers)
if response.status_code == 200:
artifacts = response.json()
print(artifacts)
else:
print(f"Error: {response.status_code}")
Example response:
{
"total": 2,
"next": null,
"prev": null,
"limit": 10,
"offset": 0,
"data": [
{
"id": "f468f3e5-f2bf-46e1-a06a-94e35f51df92",
"time_created": "2023-03-15T14:10:28.351963Z",
"time_expiration": "2023-03-22T14:10:28.351716Z",
"filename": "log-03-15-2023-14-10-27.txt",
"mime_type": "text/plain"
},
{
"id": "8e571208-6b91-42ec-8dbf-fd8bdb1986a8",
"time_created": "2023-03-15T14:10:28.196799Z",
"time_expiration": "2023-03-22T14:10:28.196425Z",
"filename": "export_s4mnvIy.zip",
"mime_type": "application/zip"
}
]
}
GET /exports/{EXPORT_ID}/artifacts/{ARTIFACT_ID}/file/
Retrieve an artifact file
Returns: Artifact file
Example request:
curl -v -H "Authorization: Key {your-api-key}" https://api.deepva.com/api/v1/exports/{export_id}/artifacts/{artifact_id}/file
import requests
headers = {"Content-Type": "application/json",
"Authorization": f"Key {your_api_key}"}
response = requests.get(f"https://api.deepva.com/api/v1/exports/{export_id}/artifacts/{artifact_id}/file",
headers=headers)
if response.status_code == 200:
artifact = response.content
print(artifact)
else:
print(f"Error: {response.status_code}")
Postman File
If you want to test our API with the handy tool Postman, you can download a Postman project here and import it in Postman:
DeepVA API.postman_collection.json (updated 26. Aug 2020, 14:48)
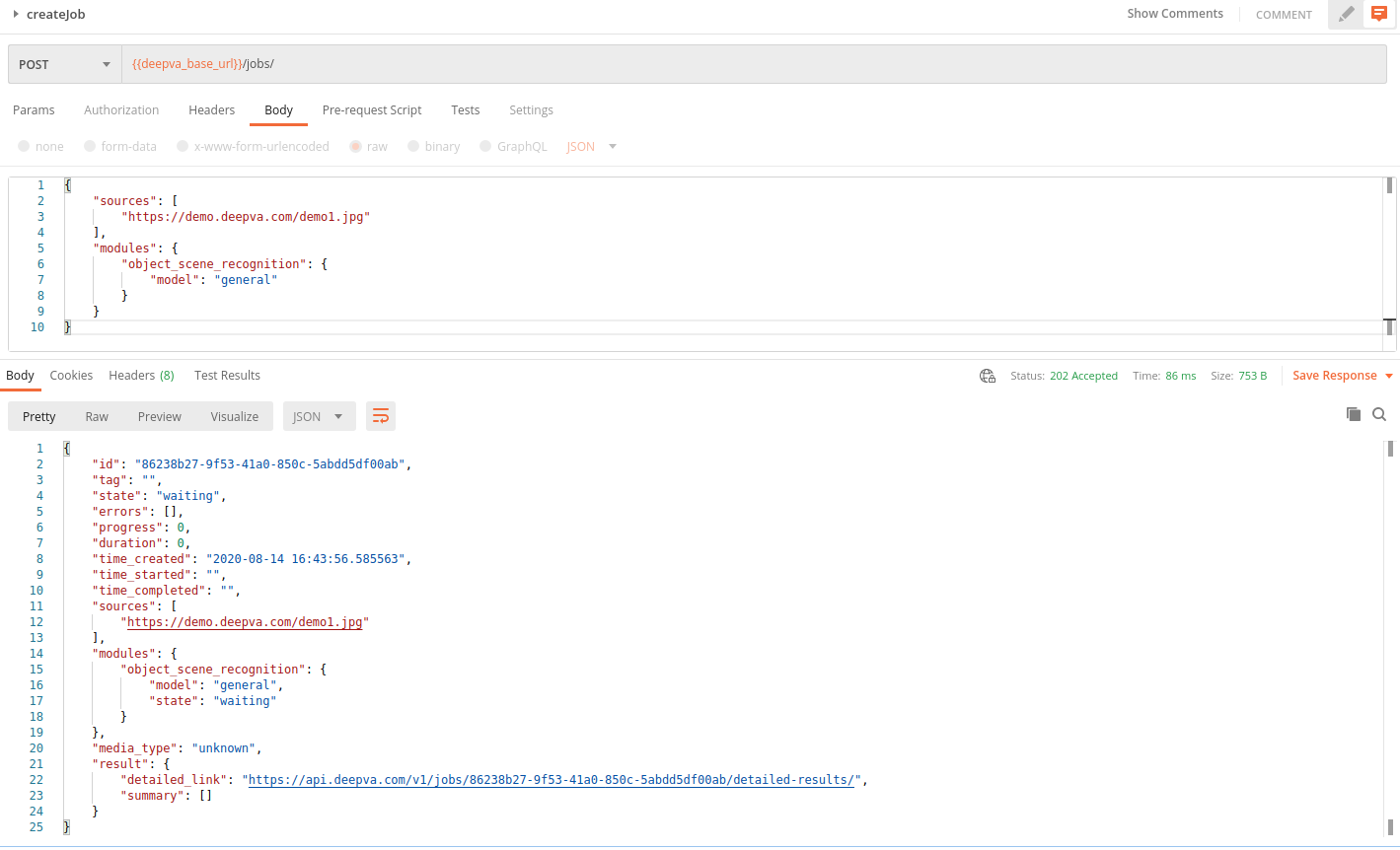